How to Allow Users to Log in to Your Web3 App With Email (Using Rainbowkit or wagmi and Paper)
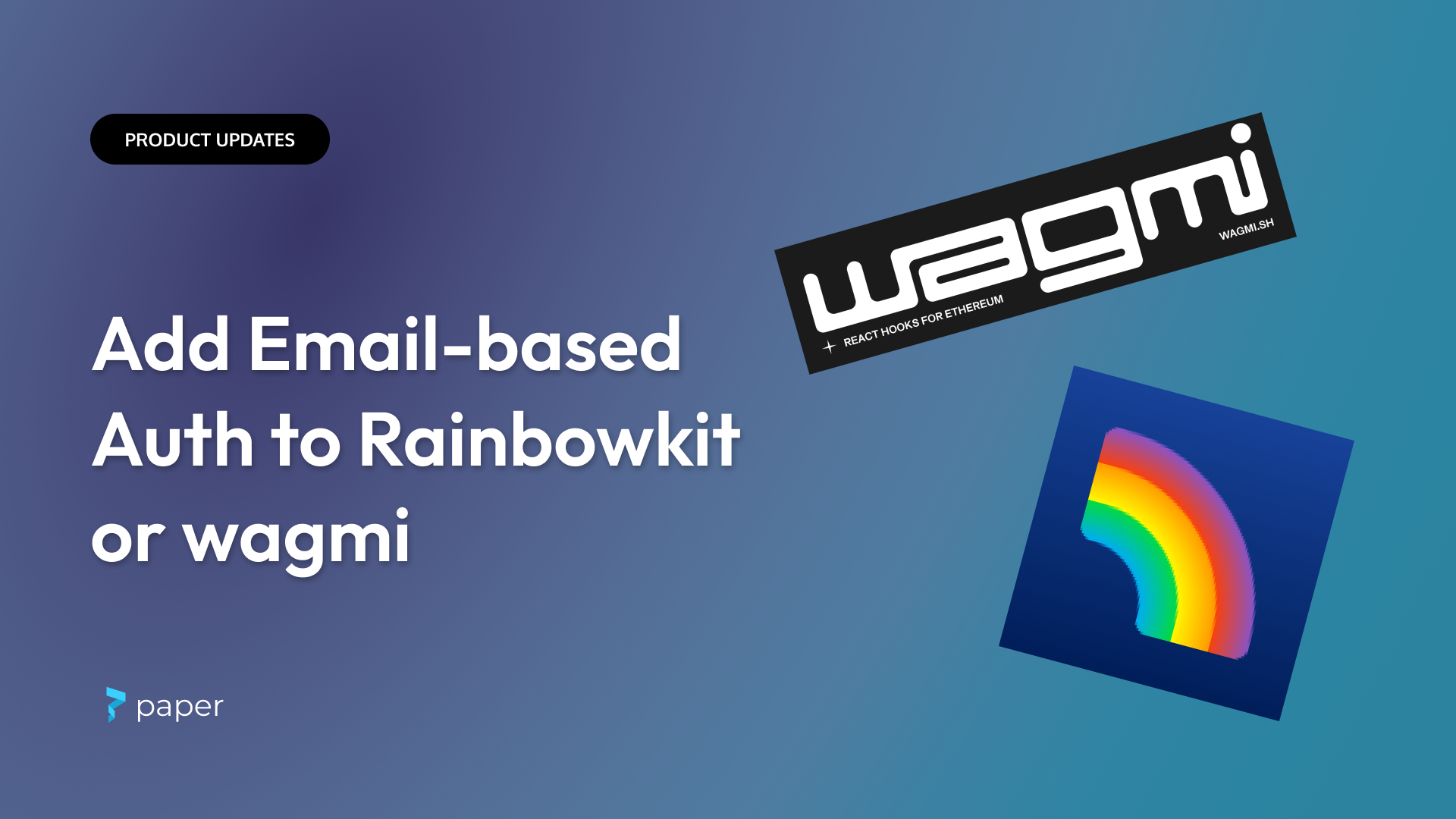
This guide will show you how to allow users to log in to your web3 app using their email in two simple steps. This guide shows you how to do that with Paper and RainbowKit or Wagmi.
What Is Paper?
Paper’s Embedded Wallets are the easiest way to log in to your web3 app using their email. It works by spinning up a non-custodial wallet tied to their email address. In other words, it allows for account abstraction using Externally Owned Account (EOA) wallets.
How to Integrate Paper with RainbowKit
What Is RainbowKit?
RainbowKit is a React library that makes it easy to add wallet connection to your dapp. It's intuitive, responsive and customizable.
RainbowKit’s Connect Wallet Button
The Connect Wallet Button is the easiest way to let users sign in to your web3 app with email or web3 wallets directly.
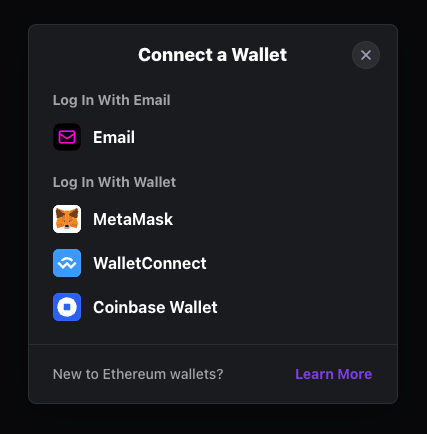
The Integration
To add email based auth, wrap your app in a Provider
and add ConnectButton
to add a sign-in button.
import {
ConnectButton,
PaperEmbeddedWalletProvider,
} from "@paperxyz/embedded-wallet-service-rainbowkit";
import "@rainbow-me/rainbowkit/styles.css";
<PaperEmbeddedWalletProvider
appName="My web3 app"
walletOptions={{
clientId: "xxxxxxxx-xxxx-xxxx-xxxxxxxxxxxx",
chain: "Polygon",
}}
>
<ConnectButton />
</PaperEmbeddedWalletProvider>
How to Customize RainbowKit
RainbowKit is fully customizable, meaning you can alter the look and feel of the button and popup, change wallet options, and more.
Read more: Embedded Wallets - Connect Wallet Button
How to Integrate Paper with wagmi
What Is wagmi?
wagmi is similar to RainbowKit.
wagmi is a collection of React Hooks containing everything you need to start working with Ethereum. wagmi makes it easy to "Connect Wallet," display ENS and balance information, sign messages, interact with contracts, and much more — all with caching, request deduplication, and persistence.
The Integration
If your React app is already using wagmi, it's simple to add Paper’s Embedded Wallets as a wallet option. This wallet provides an ethers.js-compatible Signer, meaning the extensive capabilities of wagmi work out of the box.
Your app probably has most of the code already done. Just add a new wagmi connector:
const { chains, provider } = configureChains(
[polygon],
[publicProvider()]
);
// Create a wagmi-compatible connector:
const paperEmbeddedWallet = new PaperEmbeddedWalletWagmiConnector({
chains,
options: {
chain: 'Polygon',
clientId: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
}
});
const client = createClient({
connectors: [ paperEmbeddedWallet ],
provider,
});
function App() {
return (
<WagmiConfig client={client}>
// ...your app
</WagmiConfig>
);
}
And of course, this is fully compatible with your existing wagmi code!
const { address } = useAccount();
console.log( address ? `Connected: ${address}` : 'Please sign in');
Read more: Embedded Wallets - Wagmi Connector
How to Get Your 'clientID'
Sign up for Paper here. And navigate to your Dashboard.