How to Price Your NFTs in USDC
Learn how to accept USDC as payment in your smart contract and how to withdraw your balance of USDC from your smart contract.
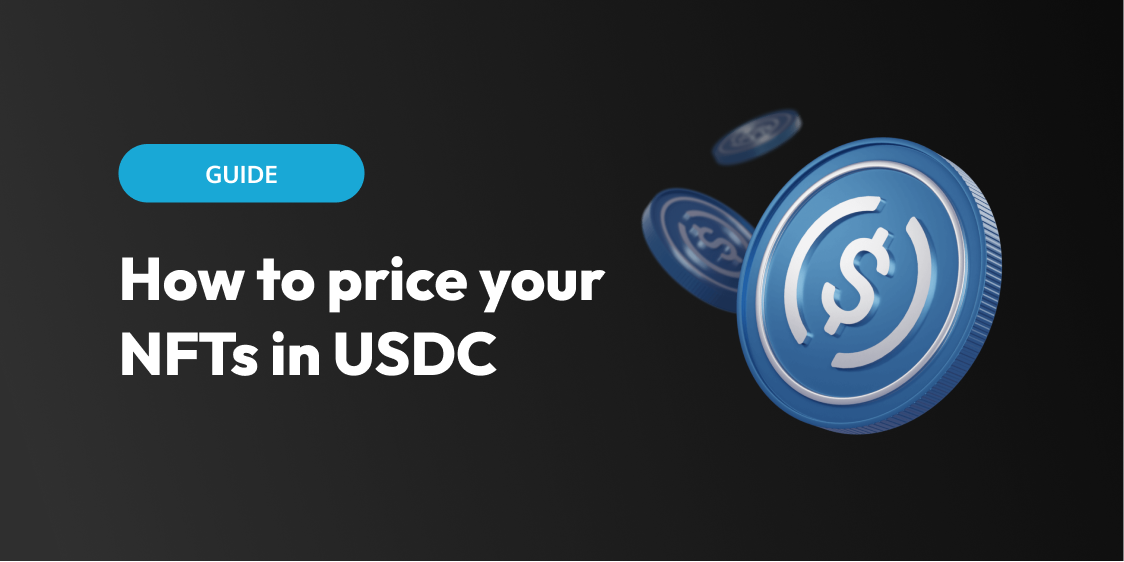
When creating an NFT Drop, one of the main things you’ll need to decide on is the price of your NFTs. Given the ever-fluctuating prices of native cryptocurrencies such as Ethereum, MATIC, Avalanche, Solana, etc., it can be really difficult to commit to a price. This is where stablecoins come in! Stablecoins are a special type of cryptocurrency whose value is pegged to a more stable asset. A really popular example of a stablecoin is USDC, which is an ERC-20 token tied to the US Dollar. This means an NFT costing $25 USDC will be equivalent in price to $25 USD!
This is pretty neat because now neither you nor your NFT buyers need to worry about the value of your NFT fluctuating. Pricing in USDC also helps your customer understand exactly how much they are paying in a measurement they natively understand, like US Dollars.
Step 1: Choose the right USDC token address
USDC is a type of ERC-20 token. This means we need to find the token address that represents USDC on the blockchain you'll be using. Remember that ERC-20 token addresses differ between testnet and mainnet. Here are common token addresses:
- USDC on Mumbai: 0xe6b8a5cf854791412c1f6efc7caf629f5df1c747
- USDC on Polygon: 0x2791bca1f2de4661ed88a30c99a7a9449aa84174
- USDC on Goerli: 0x07865c6E87B9F70255377e024ace6630C1Eaa37F
- USDC on Ethereum: 0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48
Save this address to use in Step 2!
Step 2: Accept USDC as payment in your smart contract
To price your NFTs in USDC, you’ll need to make some minor tweaks to your smart contract. If you don’t have a smart contract yet, feel free to clone this sample contract I made for a typical ERC-721 NFT Drop. Your smart contract probably has a mint function that looks like this:
contract NFT is ERC721, PullPayment, Ownable {
using Counters for Counters.Counter;
Counters.Counter private currentTokenId;
constructor() ERC721("NFTTutorial", "NFT") {}
function mintTo(address recipient) public payable {
uint256 tokenId = currentTokenId.current();
currentTokenId.increment();
_safeMint(recipient, newItemId);
}
In Solidity, to accept ERC-20 tokens such as USDC as forms of payment, you need to explicitly transfer the fixed price amount in USDC from the wallet address calling the mintTo
function. We can do this by using the transferFrom
function to transfer the amount of priceInWei
from msg.sender
to address(this)
.
msg.sender
is the wallet address of the user calling the mintTo
method. address(this)
is the address of this smart contract, which will be receiving the funds in the form of USDC ERC-20 tokens. Upon doing this, we can remove the payable
keyword in the mint method.
contract NFT is ERC721, PullPayment, Ownable {
using Counters for Counters.Counter;
Counters.Counter private currentTokenId;
IERC20 public usdc;
uint256 priceInWei = 1 * 10 ** 6
constructor(address usdcAddress) ERC721("NFTTutorial", "NFT") {
usdc = IERC20(usdcAddress);
}
function mintTo(address recipient) public {
usdc.transferFrom(msg.sender, address(this), priceInWei);
uint256 tokenId = currentTokenId.current();
currentTokenId.increment();
_safeMint(recipient, newItemId);
}
Note that, in this implementation, we are accepting the token address we chose in Step 1 as an input of the constructor of this smart contract. The token address represents the USDC ERC-20 token on the chain on you chose on Step 1.
Step 3: Withdraw USDC from your smart contract
At some point after releasing your NFT collection, you will start to accrue a balance of USDC tokens in your smart contract. Creating a withdraw method will allow you to cash out your USDC balance from your smart contract into a wallet of your choosing.
Here’s a very simple implementation of this that uses the transfer
method from the IERC-20 interface. It takes the entire balance of the smart contract and transfers it to msg.sender
, which is the wallet address of the user that is calling this method.
function withdrawToken() public onlyOwner {
usdc.transfer(msg.sender, usdc.balanceOf(address(this)));
}
Next Steps
Now that your smart contract can accept and withdraw a USDC balance, you’re ready to start putting up your NFTs up for mint! You can follow the same steps above for any ERC-20 token. And if you’re looking to accept credit card payments (or apple pay/google pay) for your NFTs, you can check out Paper - an NFT checkout tool!
About Paper
Paper offers NFT on-ramping infrastructure to help developers, games, creators, and platforms build the best NFT checkout experiences. We offer an end-to-end checkout solution that provides both back-end payments infrastructure, the front-end UX as well as the ability to create email-based wallets. Founded in early 2022 by James Sun and Edward Sun, Paper's mission is to make NFTs more accessible and useful for everyone. To date, Paper has worked with over 2500+ developers, creators, and brands such as NYFW, Fractal, YesTheory, deadmau5, and MightyJaxx.